Banker's Algorithm
Explore Banker's Algorithm with Python code example
In this practical exploration, we will immerse ourselves in the realm of the Banker's Algorithm, a critical concept in the field of operating systems designed to address the challenges of deadlock avoidance and detection. Deadlocks can paralyze a system when processes are left waiting for resources held by others, leading to a standstill. The Banker's Algorithm is our solution to managing resource allocation intelligently, preventing deadlocks, and detecting potential deadlock scenarios.
Objective of Banker's Algorithm:
The primary objectives of the Banker's Algorithm are twofold. First, it aims to ensure Deadlock Avoidance by allocating resources to processes in a manner that guarantees deadlock never occurs. This involves analyzing resource requests meticulously to assess their safety for the system's stability. Secondly, it provides a mechanism for Deadlock Detection by periodically examining resource allocation and request statuses to identify processes on the brink of causing a deadlock.
Logic and Rules of the Banker's Algorithm:
The Banker's Algorithm operates on a set of fundamental principles:
1. Processes and Resources: It defines the system in terms of processes and resource types, tracking the maximum demand of each process for each resource, current resource allocation, and the remaining resource needs.
2. Request Validation: When a process requests resources, the algorithm checks if the request satisfies key conditions: it should not exceed the process's maximum demand and must be within the available resources.
3. Safety Algorithm: The heart of the Banker's Algorithm is the safety algorithm, which determines if a sequence of resource allocations is safe. It iteratively checks if processes can safely complete their execution by assessing whether their resource needs are met by the available resources.
4. Resource Allocation: When a process is deemed safe to proceed, the algorithm allocates the required resources, updates the available resources, and marks the process as finished.
Implementing Banker's Algorithm
Now, let's implement the Banker's Algorithm in Python, step by step, using a practical example.
Example:
Consider a system with 5 processes (P0, P1, P2, P3, P4) and 3 types of resources (R0, R1, R2). We'll represent the maximum demand of each process and the current allocation of resources as matrices. The available resources vector shows the number of available instances of each resource type in the system.
Process | Max Demand(Max) | Allocation(Alloc) | Need (Max-Alloc) |
---|---|---|---|
P0 | 7,5,3 | 0,1,0 | 7,4,3 |
P1 | [3,2,2] | [2,0,0] | [1,2,2] |
P2 | [9,0,2] | [3,0,2] | [6,0,0] |
P3 | [2,2,2] | [2,1,1] | [0,1,1] |
P4 | [4,3,3] | [0,0,2] | [4,3,1] |
Available Resources: [3, 3, 2]
Banker's Algorithm Logic (Using Symbols)
Symbols Used:
- `P[i]`: Represents process `i`.
- `R[j]`: Represents resource `j`.
- `Max[i][j]`: Maximum demand of process `i` for resource `j`.
- `Alloc[i][j]`: Amount of resource `j` currently allocated to process `i`.
- `Need[i][j]`: Remaining resource need of process `i` for resource `j`.
- `Avail[j]`: Number of available instances of resource `j` in the system.
- `Work[j]`: A vector representing the available resources for each resource `j`.
- `Finish[i]`: A boolean indicating whether process `i` has finished or not.
- `SafeSequence`: An ordered list of processes indicating a safe sequence.
Initialization:
1. Initialize `Work = Available` and `Finish[i] = False` for all `i`.
Safety Algorithm:
2. While there exists an `i` such that `Finish[i] = False` and `Need[i] <= Work`, do the following:
- Set `Work = Work + Alloc[i]`.
- Set `Finish[i] = True`.
- Add `P[i]` to the end of `SafeSequence`.
3. If all `Finish[i]` are `True` for all processes, the system is in a safe state, and `SafeSequence` represents a safe execution sequence.
Resource Request Logic:
4. When a process `P[i]` requests resources `Request`, check if the request is valid:
- If `Request <= Need[i]` and `Request <= Available`, proceed to step 5.
- Otherwise, deny the request as it cannot be granted safely.
5. Temporarily allocate the requested resources to `P[i]`:
- `Work = Work - Request`
- `Alloc[i] = Alloc[i] + Request`
- `Need[i] = Need[i] - Request`
6. Check if the system remains in a safe state:
- If it does, grant the request and update `Available`.
- If it doesn't, deny the request, roll back changes, and keep the system's state unchanged.
Example:
Consider the example with 5 processes (P0, P1, P2, P3, P4) and 3 types of resources (R0, R1, R2) described in the previous table. We have the following state:
Available Resources: [3, 3, 2]
- `Max` matrix defines the maximum demand of each process for each resource.
- `Alloc` matrix represents the resources currently allocated to each process.
- `Need` matrix represents the remaining resource need for each process.
- `Available` vector shows the number of available instances of each resource type in the system.
Now, let's walk through the Banker's Algorithm logic using symbols based on this example:
- Initialization:
- Initialize `Work = Available`.
- Initialize `Finish[i] = False` for all `i`.
- Safety Algorithm:
- Check if there exists a process `P[i]` such that `Finish[i] = False` and `Need[i] <= Work`.
- If found, update `Work`, set `Finish[i] = True`, and add `P[i]` to the `SafeSequence`.
- Repeat until all processes are finished or no eligible processes are found.
- Resource Request Logic:
- When a process `P[i]` requests resources `Request`:
- Check if `Request <= Need[i]` and `Request <= Available`.
- If valid, temporarily allocate resources to `P[i]`.
- Check if the system remains in a safe state:
- If safe, grant the request and update `Available`.
- If not safe, deny the request and keep the system's state unchanged.
This logic ensures that resources are allocated in a manner that avoids deadlocks and maintains system safety.
Advantages and Disadvantages:
Advantages:
- Effective Deadlock Avoidance: The Banker's Algorithm excels at preventing deadlocks by careful resource allocation.
- Resource Efficiency: It ensures resources are allocated efficiently, maximizing system utilization.
- Real-World Relevance: This algorithm is highly relevant in real-world operating systems, enhancing system reliability.
Disadvantages:
- Conservative: The algorithm can be cautious, potentially underutilizing resources.
- Information Requirements: It relies on accurate knowledge of process resource demands, which may not always be available.
- Limited to Predefined Maximums: It assumes knowledge of maximum resource needs, which may not be feasible in dynamic systems.
Conclusion:
In concluding this, we've embarked on a journey into the world of the Banker's Algorithm, a vital tool in the realm of operating systems. We've learned about its core principles, resource allocation strategies, and its role in preventing and detecting deadlocks. Through practical simulation, we've seen how it evaluates and handles resource requests, prioritizing system safety. The Banker's Algorithm stands as a cornerstone for robust system design, ensuring resources are allocated judiciously to prevent the ominous specter of deadlocks. With a profound understanding of this algorithm, we empower ourselves to design resilient systems that operate seamlessly, free from the shackles of deadlocks.
Related Labs
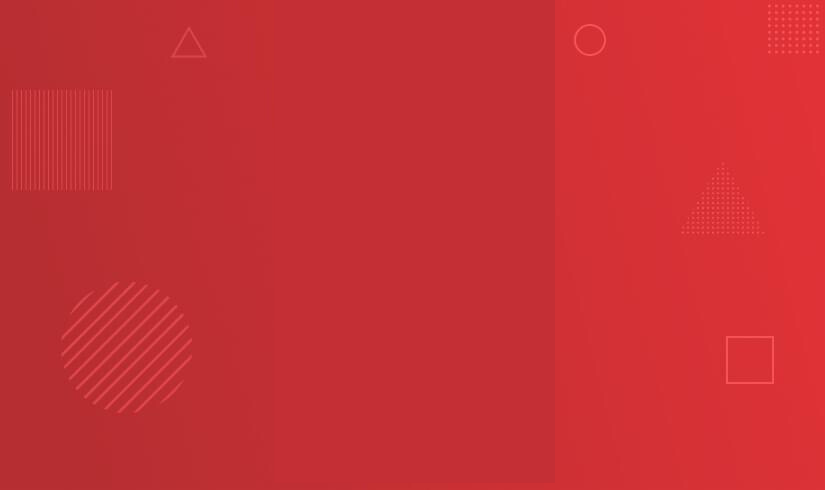
Linux Basic Commands
Operating System
- 45 m
- Beginner
- 252
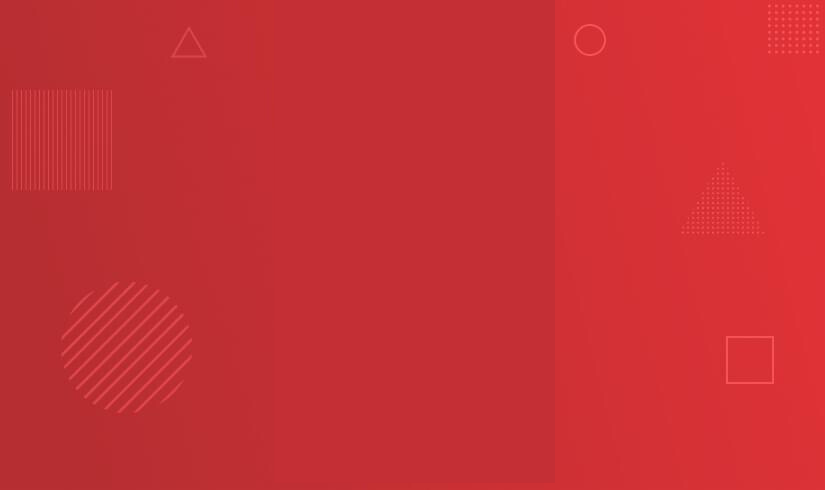
Linux System Calls
Operating System
- 30 m
- Beginner
- 274
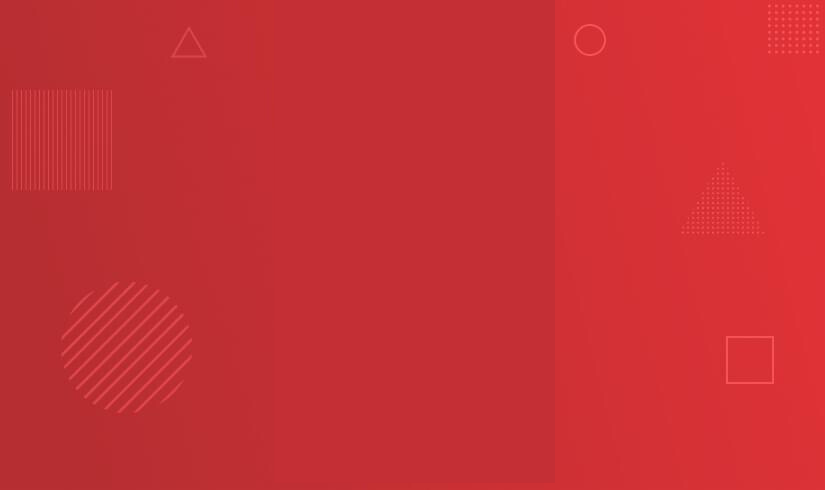
Linux I/O System Calls
Operating System
- 30 m
- Beginner
- 194