Threads
Explore fundamental concept of threads in OS with Python code
Welcome to the Threads Lab, where we delve into the fundamental concept of threads in concurrent programming. Threads, lightweight processes within a program, allow for simultaneous execution of tasks, enhancing efficiency and responsiveness. Throughout this lab, we'll explore the creation, lifecycle, types, and challenges associated with threads, empowering you with valuable insights into this critical aspect of software development.
What are threads?
Threads are a fundamental concept in computer science and operating systems. They represent the smallest unit of execution within a process. A process, in turn, is an instance of a program running on a computer.
Threads within a process share the same memory space, allowing them to communicate and cooperate more efficiently than separate processes. Threads can be thought of as parallel paths of execution within a program. They allow a program to perform multiple tasks concurrently, thus improving performance and responsiveness.
Threads have their execution stack but share memory and other resources such as file descriptors, sockets, and other process-related states with other threads in the same process. This shared memory model allows threads to communicate and synchronize their activities, but it also requires careful management to avoid issues like race conditions and deadlocks.
Threads are commonly used in applications where responsiveness and concurrency are important, such as graphical user interfaces, web servers, and multimedia processing software. They enable tasks to be performed simultaneously, leading to better resource utilization and faster execution times.
Threads vs Process
-
Processes are independent units with separate memory spaces, while threads are lighter-weight units within a process sharing the same memory space.
-
Processes incur higher overhead during creation and context switching compared to threads.
-
Threads allow for more efficient communication and synchronization due to their shared context.
-
Processes offer better fault isolation, as failure in one process typically doesn't affect others, while threads within the same process may interfere with each other.
-
The choice between threads and processes depends on factors such as resource utilization, fault isolation requirements, and parallelism needs.
Types of Threads
Threads can be categorized into two main types based on their concurrency model: single-threaded and multi-threaded.
-
Single-threaded:
-
Single-threaded refers to programs that execute only one thread of control. In other words, they have only one sequence of execution.
-
These programs are straightforward in design and execution because there's only one path of execution to follow.
-
Single-threaded programs are inherently sequential, meaning that tasks are executed one after the other.
-
They are commonly used in simple applications or scenarios where concurrency is not necessary or where the overhead of managing multiple threads outweighs any potential benefits.
-
Multi-threaded:
-
Multi-threaded programs have multiple threads of control, meaning they can execute multiple tasks concurrently.
-
Each thread in a multi-threaded program represents a separate path of execution that can run simultaneously with other threads within the same process.
-
Multi-threaded programs can achieve greater responsiveness and improved performance by parallelizing tasks and utilizing available system resources more efficiently.
-
However, managing multiple threads introduces challenges such as synchronization, race conditions, and deadlock avoidance.
-
Multi-threading is commonly used in applications where concurrency is essential, such as web servers, graphical user interfaces, multimedia processing, and parallel computing tasks.
Lifecycle of Threads:
-
Creation: Threads are created with unique identifiers and necessary resources.
-
Ready/Runnable: Threads are ready to execute but awaiting CPU scheduling.
-
Running: Execution of thread instructions on the CPU.
-
Blocked/Waiting: Threads are waiting for external events or resources.
-
Termination: Threads end execution and release allocated resources.
Threading Issues:
-
Concurrency Control: Coordinating thread access to shared resources.
-
Deadlocks: Threads are blocked waiting for resources held by each other.
-
Starvation: Threads are continuously denied access to resources.
-
Thread Safety: Ensuring data structures can be accessed safely by multiple threads.
-
Performance Overhead: Overhead from context switching, synchronization, and coordination.
-
Debugging and Testing: Challenges in debugging and testing due to non-deterministic behavior.
Conclusion
In conclusion, understanding the lifecycle of threads and addressing threading issues are fundamental aspects of developing reliable and efficient multi-threaded applications. The lifecycle stages, from creation to termination, outline the journey of a thread within a process, while threading issues such as concurrency control, deadlocks, and performance overhead highlight the challenges developers face in ensuring thread safety and optimal performance. By mastering techniques for managing thread execution, synchronizing access to shared resources, and debugging multi-threaded code, developers can create robust applications capable of leveraging the benefits of parallelism and concurrency while mitigating potential pitfalls and ensuring overall system stability and reliability.
Threads
Related Labs
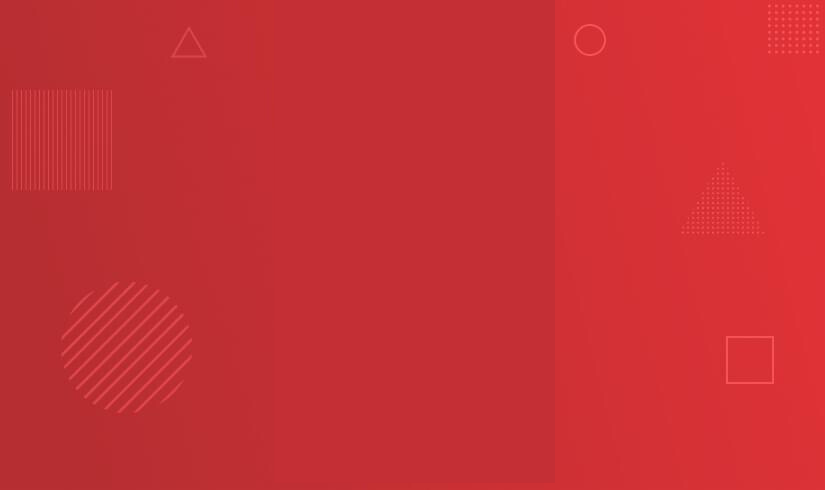
Linux Basic Commands
Operating System
- 45 m
- Beginner
- 252
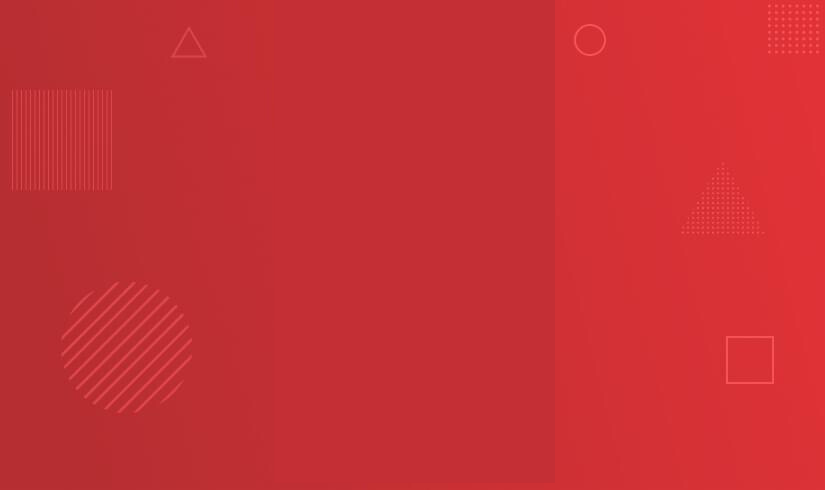
Linux System Calls
Operating System
- 30 m
- Beginner
- 274
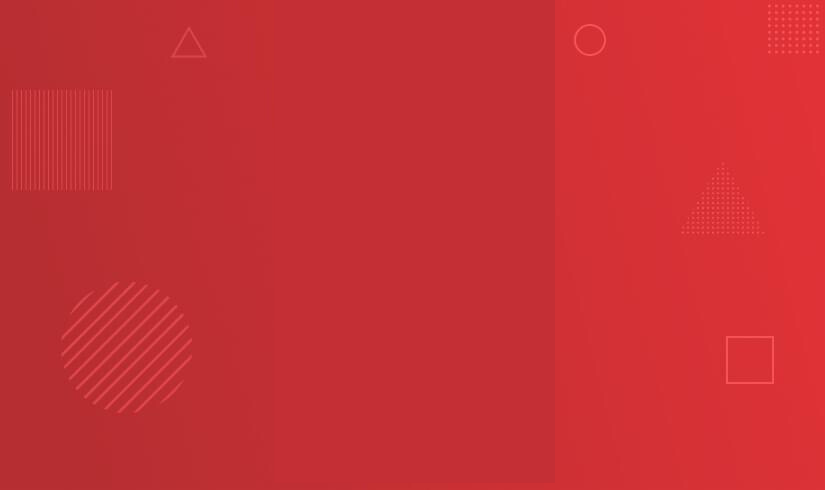
Linux I/O System Calls
Operating System
- 30 m
- Beginner
- 194